Scheduled reports
Send reports on a periodic basis to your users, at a frequency chosen by them.
To generate a report, your users will click on the reports button on the top right toolbar of the dashboard.
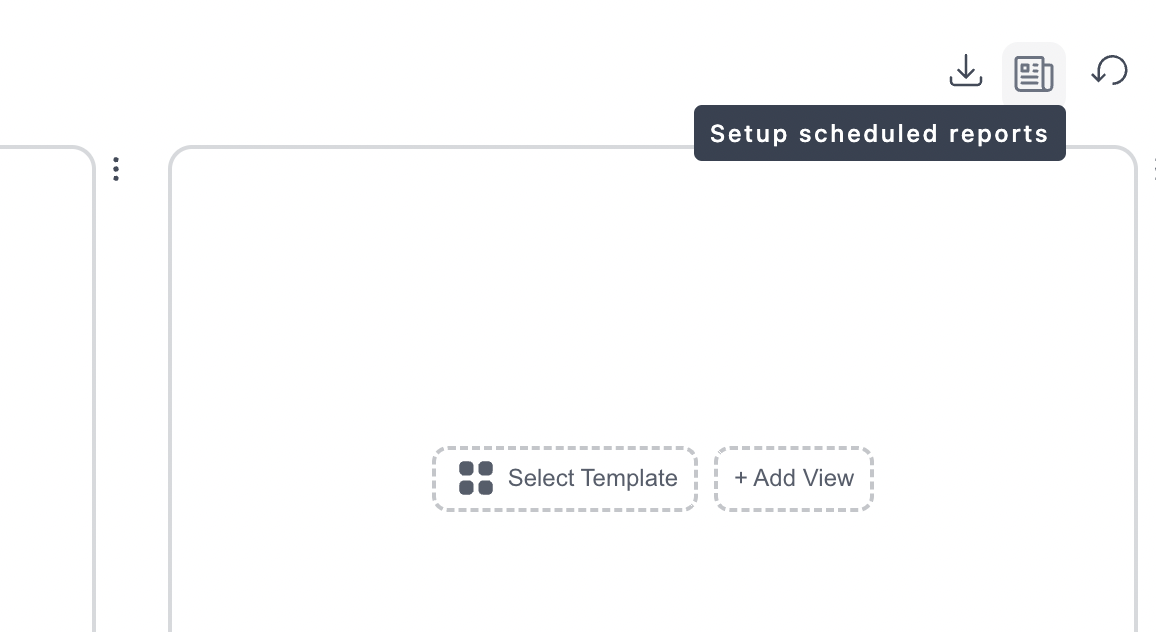
Vizzly manages the scheduling of webhooks, and sends your servers an HTTP request when each user requires their report.
Once you have enabled webhooks for a dashboard, you can add the property reportScheduleOptions
to the Vizzly Dashboard component.
which would allow your users to pick from an "every minute", or an "every hour" schedule.
The webhook event
The body of the POST
request you will receive is a JWT, which contains a payload of the webhook event data.
The JWT should be verified using the Vizzly webhook public keys, which can be fetched from the Vizzly API at these URLs;
The public keys are base64 encoded, and once decoded, they can be used to verify the webhook event.